Java应用程序性能调优和内存管理
上次更新时间:2024-12-01
课程售价: 2.9 元
联系右侧微信客服充值或购买课程
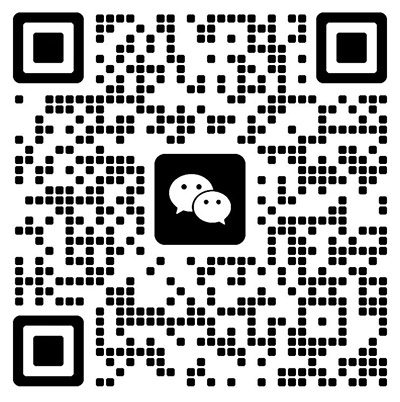
课程内容
01 - Chapter 1 - Introduction
02 - Chapter 2 - Just In Time Compilation and the Code Cache
- 001 What is bytecode
- 002 The concept of Just In Time Compilation
- 003 Introducing the first example project
- 004 Finding out which methods are being compiled in our applications
- 005 The C1 and C2 Compilers and logging the compilation activity
- 006 Tuning the code cache size
- 007 Remotely monitoring the code cache with JConsole
03 - Chapter 3 - Selecting the JVM
04 - Chapter 4 - How memory works - the stack and the heap
05 - Chapter 5 - Passing objects between methods
06 - Chapter 6 - Memory exercise 1
07 - Chapter 7 - Escaping References
- 001 Introduction - what is an escaping reference
- 002 Strategy 1 - using an iterator
- 003 Strategy 2 - duplicating collections
- 004 Strategy 3 - using immutable collections
- 005 Strategy 4 - duplicating objects
- 006 Strategy 5 - using interfaces to create immutable objects
- 007 Strategy 6 - using modules to hide the implementation
08 - Chapter 8 - Memory Exercise 2
09 - Chapter 9 - The Metaspace and internal JVM memory optimisations
10 - Chapter 10 - Tuning the JVM's Memory Settings
11 - Chapter 11 - Introducing Garbage Collection
- 001 What it means when we say Java is a managed language
- 002 How Java knows which objects can be removed from the Heap
- 003 The System.gc() method
- 004 Java 11's garbage collector can give unused memory back to the operating system
- 005 Why it's not a good idea to run the System.gc() method
- 006 The finalize() method
- 007 The danger of using finalize()
12 - Chapter 12 - Monitoring the Heap
13 - Chapter 13 - Analysing a heap dump
14 - Chapter 14 - Generational Garbage Collection
15 - Chapter 15 - Garbage Collector tuning & selection
- 001 Monitoring garbage collections
- 002 Turning off automated heap allocation sizing
- 003 Tuning garbage collection - old and young allocation
- 004 Tuning garbage collection - survivor space allocation
- 005 Tuning garbage collection - generations needed to become old
- 006 Selecting a garbage collector
- 007 The G1 garbage collector
- 008 Tuning the G1 garbage collector
- 009 String de-duplication
16 - Chapter 16 - Using a profiler to analyse application performance
- 001 Introducing Java Mission Control (JMC)
- 002 Building the JMC binaries
- 003 Running JMC and connecting to a VM
- 004 Customising the overview tab
- 005 The MBean Browser tab
- 006 The System, Memory and Diagnostic Commands tabs
- 007 Introducing our problem project
- 008 Using the flight recorder
- 009 Analyzing a flight recording
- 010 Improving our application
17 - Chapter 17 - Assessing Performance
18 - Chapter 18 - Benchmarking with JMH
19 - Chapter 19 - Performance and Benchmarking Exercise
- 001 Instructions for exercise 1 (creating a flight recording)
- 002 Walkthrough of the solution & setting up ready for the next challenge
- 003 Instructions for exercise 2 (use JMH to macrobenchmark the project)
- 004 Walkthrough of the solution - part 1 setting up the code
- 005 Walkthrough of the solution - part 2 - integrating into JMH
20 - Chapter 20 - How Lists Work
- 001 Why it's important to understand how the different List implementations work
- 002 The 8 different list implementations
- 003 The CopyOnWriteArrayList
- 004 The ArrayList
- 005 Specifying the initial size of an ArrayList
- 006 The Vector
- 007 The Stack
- 008 The LinkedList
- 009 Choosing the optimal list type
- 010 Sorting lists
21 - Chapter 21 - How Maps Work
- 001 How Hashmaps Work - part 1
- 002 The role of the Hashcode
- 003 How Hashmaps Work - part 2
- 004 Specifying the initial size and factor of a HashMap
- 005 HashMap Performance
- 006 The rules for Hashcodes
- 007 Generating and optimising the Hashcode method
- 008 Optimising Hashmap Performance
- 009 How The LinkedHashMap Works
- 010 The HashTable and TreeMap
22 - Chapter 22 - Other Coding Choices
23 - Chapter 23 - GraalVM
24 - Chapter 24 - Using Other JVM Languages
25 - Chapter 25 - Course Summary
课程内容
25个章节 , 133个讲座
01 - Chapter 1 - Introduction
02 - Chapter 2 - Just In Time Compilation and the Code Cache
- 001 What is bytecode
- 002 The concept of Just In Time Compilation
- 003 Introducing the first example project
- 004 Finding out which methods are being compiled in our applications
- 005 The C1 and C2 Compilers and logging the compilation activity
- 006 Tuning the code cache size
- 007 Remotely monitoring the code cache with JConsole
03 - Chapter 3 - Selecting the JVM
04 - Chapter 4 - How memory works - the stack and the heap
05 - Chapter 5 - Passing objects between methods
06 - Chapter 6 - Memory exercise 1
07 - Chapter 7 - Escaping References
- 001 Introduction - what is an escaping reference
- 002 Strategy 1 - using an iterator
- 003 Strategy 2 - duplicating collections
- 004 Strategy 3 - using immutable collections
- 005 Strategy 4 - duplicating objects
- 006 Strategy 5 - using interfaces to create immutable objects
- 007 Strategy 6 - using modules to hide the implementation
08 - Chapter 8 - Memory Exercise 2
09 - Chapter 9 - The Metaspace and internal JVM memory optimisations
10 - Chapter 10 - Tuning the JVM's Memory Settings
11 - Chapter 11 - Introducing Garbage Collection
- 001 What it means when we say Java is a managed language
- 002 How Java knows which objects can be removed from the Heap
- 003 The System.gc() method
- 004 Java 11's garbage collector can give unused memory back to the operating system
- 005 Why it's not a good idea to run the System.gc() method
- 006 The finalize() method
- 007 The danger of using finalize()
12 - Chapter 12 - Monitoring the Heap
13 - Chapter 13 - Analysing a heap dump
14 - Chapter 14 - Generational Garbage Collection
15 - Chapter 15 - Garbage Collector tuning & selection
- 001 Monitoring garbage collections
- 002 Turning off automated heap allocation sizing
- 003 Tuning garbage collection - old and young allocation
- 004 Tuning garbage collection - survivor space allocation
- 005 Tuning garbage collection - generations needed to become old
- 006 Selecting a garbage collector
- 007 The G1 garbage collector
- 008 Tuning the G1 garbage collector
- 009 String de-duplication
16 - Chapter 16 - Using a profiler to analyse application performance
- 001 Introducing Java Mission Control (JMC)
- 002 Building the JMC binaries
- 003 Running JMC and connecting to a VM
- 004 Customising the overview tab
- 005 The MBean Browser tab
- 006 The System, Memory and Diagnostic Commands tabs
- 007 Introducing our problem project
- 008 Using the flight recorder
- 009 Analyzing a flight recording
- 010 Improving our application
17 - Chapter 17 - Assessing Performance
18 - Chapter 18 - Benchmarking with JMH
19 - Chapter 19 - Performance and Benchmarking Exercise
- 001 Instructions for exercise 1 (creating a flight recording)
- 002 Walkthrough of the solution & setting up ready for the next challenge
- 003 Instructions for exercise 2 (use JMH to macrobenchmark the project)
- 004 Walkthrough of the solution - part 1 setting up the code
- 005 Walkthrough of the solution - part 2 - integrating into JMH
20 - Chapter 20 - How Lists Work
- 001 Why it's important to understand how the different List implementations work
- 002 The 8 different list implementations
- 003 The CopyOnWriteArrayList
- 004 The ArrayList
- 005 Specifying the initial size of an ArrayList
- 006 The Vector
- 007 The Stack
- 008 The LinkedList
- 009 Choosing the optimal list type
- 010 Sorting lists
21 - Chapter 21 - How Maps Work
- 001 How Hashmaps Work - part 1
- 002 The role of the Hashcode
- 003 How Hashmaps Work - part 2
- 004 Specifying the initial size and factor of a HashMap
- 005 HashMap Performance
- 006 The rules for Hashcodes
- 007 Generating and optimising the Hashcode method
- 008 Optimising Hashmap Performance
- 009 How The LinkedHashMap Works
- 010 The HashTable and TreeMap