使用 C 和数据结构进行编程(从基础到高级)
上次更新时间:2024-11-16
课程售价: 2.9 元
联系右侧微信客服充值或购买课程
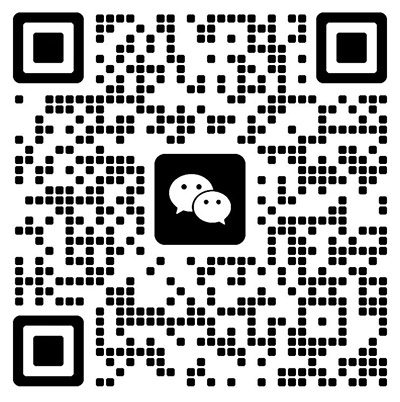
课程内容
- 1 - Why Programming (免费)
- 2 - History of C Language (免费)
- 3 - Hello World Program
- 4 - Identifiers in C
- 5 - Variables and DataTypes
- 6 - Constants
- 7 - Printf and Scanf
- 8 - Unformatted IO Functions
- 9 - Expressions and Arithmetic Operators
- 10 - Relational and Logical Operators
- 11 - Bitwise Operators
- 12 - Unconditional Branching using goto statement
- 13 - If Statement
- 14 - Switch Statement
- 15 - While Loop
- 16 - Do While Loop
- 17 - For Loop
- 18 - Break and Continue
- 19 - Special Cases
- 20 - Introduction and Writing Functions
- 21 - Scope of VariablesStorage ClassesPass by Value and reference
- 22 - Recursion
- 23 - Arrays Declaration and Initialization
- 24 - Sample Programs using Arrays
- 25 - Arrays as Function Parameters
- 26 - 2Dimensional Array
- 27 - Introduction to Pointers
- 28 - Pointers as Function Parameter
- 29 - Pointer Arithmetic
- 30 - Function Pointers
- 31 - Dynamic Memory Allocation using malloc
- 32 - calloc and comparision with malloc
- 33 - Introduction to Strings
- 34 - Sample Program
- 35 - More Sample Programs
- 36 - Standard String Library Functions
- 37 - Array of String
- 38 - Declaring and Instantiating Structures
- 39 - Structure as Parameter and Pointer to Structure
- 40 - Enumerated Data Type
- 41 - Union
- 42 - Bit Fields
- 43 - What is a Stream
- 44 - File HandlingWriting and Reading Characters
- 45 - Writing and Reading Structure in Text Format
- 46 - Writing and Reading in Binary Format
- 47 - Understanding PreProcessor directives
- 48 - Header Files and Project
- 49 - Command Line Argument
- 50 - Variable Number of Arguments
- 51 - Linear Search
- 52 - Iterative Binary Search
- 53 - Recursive Binary Search
- 54 - Bubble Sort
- 55 - Selection Sort
- 56 - Insertion Sort
- 57 - Quick Sort
- 58 - Merge Sort
- 59 - Introduction to Data Structures
- 60 - Stack Using Arrays
- 61 - Stack Using Linked List
- 62 - Infix to Postfix Expressions
- 63 - Queue Using Arrays
- 64 - Queue Using Linked List
- 65 - Introduction to Linked List
- 66 - Single Linked List
- 67 - Double Linked List
- 68 - Circular Linked List
- 69 - Introduction to Trees
- 70 - Programming Tree 1
- 71 - Programming Tree 2
- 72 - BONUS LECTURE What Next
课程内容
72个讲座
- 1 - Why Programming (免费)
- 2 - History of C Language (免费)
- 3 - Hello World Program
- 4 - Identifiers in C
- 5 - Variables and DataTypes
- 6 - Constants
- 7 - Printf and Scanf
- 8 - Unformatted IO Functions
- 9 - Expressions and Arithmetic Operators
- 10 - Relational and Logical Operators
- 11 - Bitwise Operators
- 12 - Unconditional Branching using goto statement
- 13 - If Statement
- 14 - Switch Statement
- 15 - While Loop
- 16 - Do While Loop
- 17 - For Loop
- 18 - Break and Continue
- 19 - Special Cases
- 20 - Introduction and Writing Functions
- 21 - Scope of VariablesStorage ClassesPass by Value and reference
- 22 - Recursion
- 23 - Arrays Declaration and Initialization
- 24 - Sample Programs using Arrays
- 25 - Arrays as Function Parameters
- 26 - 2Dimensional Array
- 27 - Introduction to Pointers
- 28 - Pointers as Function Parameter
- 29 - Pointer Arithmetic
- 30 - Function Pointers
- 31 - Dynamic Memory Allocation using malloc
- 32 - calloc and comparision with malloc
- 33 - Introduction to Strings
- 34 - Sample Program
- 35 - More Sample Programs
- 36 - Standard String Library Functions
- 37 - Array of String
- 38 - Declaring and Instantiating Structures
- 39 - Structure as Parameter and Pointer to Structure
- 40 - Enumerated Data Type
- 41 - Union
- 42 - Bit Fields
- 43 - What is a Stream
- 44 - File HandlingWriting and Reading Characters
- 45 - Writing and Reading Structure in Text Format
- 46 - Writing and Reading in Binary Format
- 47 - Understanding PreProcessor directives
- 48 - Header Files and Project
- 49 - Command Line Argument
- 50 - Variable Number of Arguments
- 51 - Linear Search
- 52 - Iterative Binary Search
- 53 - Recursive Binary Search
- 54 - Bubble Sort
- 55 - Selection Sort
- 56 - Insertion Sort
- 57 - Quick Sort
- 58 - Merge Sort
- 59 - Introduction to Data Structures
- 60 - Stack Using Arrays
- 61 - Stack Using Linked List
- 62 - Infix to Postfix Expressions
- 63 - Queue Using Arrays
- 64 - Queue Using Linked List
- 65 - Introduction to Linked List
- 66 - Single Linked List
- 67 - Double Linked List
- 68 - Circular Linked List
- 69 - Introduction to Trees
- 70 - Programming Tree 1
- 71 - Programming Tree 2
- 72 - BONUS LECTURE What Next