使用 Mosh 编写代码 - 终极 C Sharp 精通系列 [4 合 1]
上次更新时间:2024-11-14
课程售价: 2.9 元
联系右侧微信客服充值或购买课程
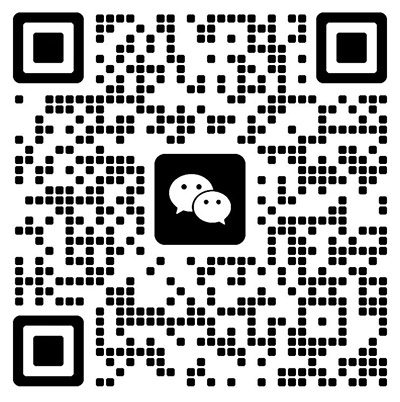
课程内容
The Ultimate C# Series - Part 1
- About This Course (免费)
- Architecture of .NET Applications (免费)
- C# vs .NET
- Introduction
- What is CLR
- Your First C# Application
- Comments
- Demo - Operators
- Demo - Type Conversion
- Demo - Variables and Constants
- Introduction
- Operators
- Overflowing
- Scope
- Summary
- Type Conversion
- Variables and Constants
- Arrays
- Classes
- Demo - Arrays
- Demo - Classes
- Demo - Enums
- Demo - Reference Types and Value Types
- Demo - Strings
- Enums
- Introduction
- Reference Types and Value Types
- Strings
- Structs
- Summary
- Conditional Statements
- Demo - For loops
- Demo - Foreach loops
- Demo - If_Else, Switch_Case
- Demo - While loops
- Introduction
- Iteration Statements
- Random Class
- Summary
- Arrays
- Demo - Arrays
- Demo - Lists
- Introduction
- Lists
- Summary
- DateTime
- Introduction
- Summary
- TimeSpan
- Demo - StringBuilder
- Demo - Strings
- Introduction
- Live Coding - Summarizing Text
- Procedural Programming
- String
- StringBuilder
- Summary
- Directory and Directory Info
- File and File Info
- Introduction
- Introduction to System.IO
- Path
- Summary
- Call Stack Window
- Debugging Tools in Visual Studio
- Defensive Programming
- Introduction
- Locals and Autos Windows
- Removing Side Effects
- Summary
- Whats Next
The Ultimate C# Series - Part 2
- About this Course
- Access Modifiers
- Constructors
- Fields
- Indexers
- Introduction to Classes
- Methods
- Object Initializers
- Properties
- Class Coupling
- Composition
- Composition over Inheritance
- Inheritance
- Access Modifiers
- Boxing and Unboxing
- Constructors and Inheritance
- Upcasting and Downcasting
- Abstract Classes and Members
- Method Overriding
- Sealed Classes and Members
- Interfaces and Extensibility
- Interfaces and Polymorphism
- Interfaces and Testability
- Interfaces are NOT for Multiple Inheritance
- What is an Interface
- Stack Exercise
- StopWatch Exercise
- WorkflowEngine Exercise
The Ultimate C# Series - Part 3
Unit Testing for C# Developers
- Benefits of Automated Testing
- Course Structure
- Refactoring with Confidence
- Source Code
- Summary
- Test Pyramid
- Testing All the Execution Paths
- The Tooling
- Types of Tests
- Using NUnit in Visual Studio
- What is Automated Testing
- What is Test-driven Development
- Writing Your First Unit Test
- Black-box Testing
- Characteristics of Good Unit Tests
- Developers Who Don't Write Tests
- Ignoring Tests
- Introducing Rider
- Introduction
- Naming and Organizing Tests
- Parameterized Tests
- Set Up and Tear Down
- Summary
- What to Test and What Not to Test
- Writing a Simple Unit Test
- Writing Trustworthy Tests
- Code Coverage
- Introduction
- Summary
- Testing Arrays and Collections
- Testing in the Real-world
- Testing Methods that Raise an Event
- Testing Methods that Throw Exceptions
- Testing Private Methods
- Testing Strings
- Testing the Return Type of Methods
- Testing Void Methods
- Exercise- DemeritPointsCalculator
- Exercise- FizzBuzz
- Exercise- Stack
- Solution- DemeritPointsCalculator
- Solution- FizzBuzz
- Solution- Stack
- An Example of a Mock Abuse
- Creating Mock Objects Using Moq
- Dependency Injection Frameworks
- Dependency Injection via Constructor
- Dependency Injection via Method Parameters
- Dependency Injection via Properties
- Fake as Little as Possible
- Introduction
- Loosely-coupled and Testable Code
- Mocking Frameworks
- Refactoring Towards a Loosely-coupled Design
- State-based vs Interaction Testing
- Testing the Interaction between Two Objects
- Who Should Write Tests
- Exercise- EmployeeController
- Exercise- InstallerHelper
- Exercise-Video Service
- Refacatroing EmployeeController
- Refactoring InstallerHelper
- Refactoring VideoService
- Testing EmployeeController
- Testing InstallerHelper
- Testing VideoService
- Extracting IBookingRepository
- Fixing a Bug
- Introduction
- Refactoring
- Test Cases
- Writing Additional Tests
- Writing the First Test
- Writing the Second Test
- Another Interaction Test
- Extracting Helper Methods
- Fixing a Design Issue
- Introduction
- Keeping Tests Clean
- Refactoring for Testability
- Testing Exceptions
- Testing that a Method is Not Called
- Writing the First Interaction Test
课程内容
4个章节 , 192个讲座
The Ultimate C# Series - Part 1
- About This Course (免费)
- Architecture of .NET Applications (免费)
- C# vs .NET
- Introduction
- What is CLR
- Your First C# Application
- Comments
- Demo - Operators
- Demo - Type Conversion
- Demo - Variables and Constants
- Introduction
- Operators
- Overflowing
- Scope
- Summary
- Type Conversion
- Variables and Constants
- Arrays
- Classes
- Demo - Arrays
- Demo - Classes
- Demo - Enums
- Demo - Reference Types and Value Types
- Demo - Strings
- Enums
- Introduction
- Reference Types and Value Types
- Strings
- Structs
- Summary
- Conditional Statements
- Demo - For loops
- Demo - Foreach loops
- Demo - If_Else, Switch_Case
- Demo - While loops
- Introduction
- Iteration Statements
- Random Class
- Summary
- Arrays
- Demo - Arrays
- Demo - Lists
- Introduction
- Lists
- Summary
- DateTime
- Introduction
- Summary
- TimeSpan
- Demo - StringBuilder
- Demo - Strings
- Introduction
- Live Coding - Summarizing Text
- Procedural Programming
- String
- StringBuilder
- Summary
- Directory and Directory Info
- File and File Info
- Introduction
- Introduction to System.IO
- Path
- Summary
- Call Stack Window
- Debugging Tools in Visual Studio
- Defensive Programming
- Introduction
- Locals and Autos Windows
- Removing Side Effects
- Summary
- Whats Next
The Ultimate C# Series - Part 2
- About this Course
- Access Modifiers
- Constructors
- Fields
- Indexers
- Introduction to Classes
- Methods
- Object Initializers
- Properties
- Class Coupling
- Composition
- Composition over Inheritance
- Inheritance
- Access Modifiers
- Boxing and Unboxing
- Constructors and Inheritance
- Upcasting and Downcasting
- Abstract Classes and Members
- Method Overriding
- Sealed Classes and Members
- Interfaces and Extensibility
- Interfaces and Polymorphism
- Interfaces and Testability
- Interfaces are NOT for Multiple Inheritance
- What is an Interface
- Stack Exercise
- StopWatch Exercise
- WorkflowEngine Exercise
The Ultimate C# Series - Part 3
Unit Testing for C# Developers
- Benefits of Automated Testing
- Course Structure
- Refactoring with Confidence
- Source Code
- Summary
- Test Pyramid
- Testing All the Execution Paths
- The Tooling
- Types of Tests
- Using NUnit in Visual Studio
- What is Automated Testing
- What is Test-driven Development
- Writing Your First Unit Test
- Black-box Testing
- Characteristics of Good Unit Tests
- Developers Who Don't Write Tests
- Ignoring Tests
- Introducing Rider
- Introduction
- Naming and Organizing Tests
- Parameterized Tests
- Set Up and Tear Down
- Summary
- What to Test and What Not to Test
- Writing a Simple Unit Test
- Writing Trustworthy Tests
- Code Coverage
- Introduction
- Summary
- Testing Arrays and Collections
- Testing in the Real-world
- Testing Methods that Raise an Event
- Testing Methods that Throw Exceptions
- Testing Private Methods
- Testing Strings
- Testing the Return Type of Methods
- Testing Void Methods
- Exercise- DemeritPointsCalculator
- Exercise- FizzBuzz
- Exercise- Stack
- Solution- DemeritPointsCalculator
- Solution- FizzBuzz
- Solution- Stack
- An Example of a Mock Abuse
- Creating Mock Objects Using Moq
- Dependency Injection Frameworks
- Dependency Injection via Constructor
- Dependency Injection via Method Parameters
- Dependency Injection via Properties
- Fake as Little as Possible
- Introduction
- Loosely-coupled and Testable Code
- Mocking Frameworks
- Refactoring Towards a Loosely-coupled Design
- State-based vs Interaction Testing
- Testing the Interaction between Two Objects
- Who Should Write Tests
- Exercise- EmployeeController
- Exercise- InstallerHelper
- Exercise-Video Service
- Refacatroing EmployeeController
- Refactoring InstallerHelper
- Refactoring VideoService
- Testing EmployeeController
- Testing InstallerHelper
- Testing VideoService
- Extracting IBookingRepository
- Fixing a Bug
- Introduction
- Refactoring
- Test Cases
- Writing Additional Tests
- Writing the First Test
- Writing the Second Test
- Another Interaction Test
- Extracting Helper Methods
- Fixing a Design Issue
- Introduction
- Keeping Tests Clean
- Refactoring for Testability
- Testing Exceptions
- Testing that a Method is Not Called
- Writing the First Interaction Test