C++ 编程语言 - 学习和掌握 C++
上次更新时间:2024-11-13
课程售价: 2.9 元
联系右侧微信客服充值或购买课程
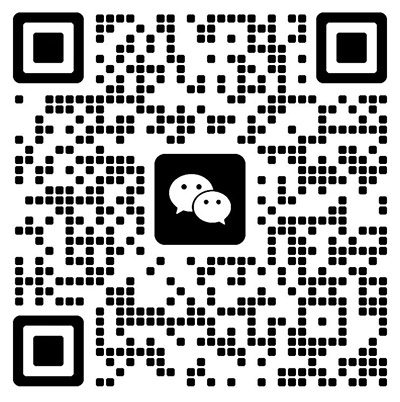
课程内容
1. Introduction
- 1. Introduction (免费)
- 2. Program Installation & Setup (Mac) (免费)
- 3. Program Installation & Setup (Windows)
- 4. Creating a C++ (.cpp) file
- 5. Hello World in C++
- 6. Overview of the C++ Programming Language
- 8. Answer and Explanation to Coding Exercise
- 9. History of C++
- 10. Running C++ Code on Mac (Terminal)
- 11. Running C++ Code on Windows (Terminal)
- 12. Key Takeaways
2. Basics of C++
- 1. Section Introduction
- 2. How is a C++ program run
- 3. #include
- 4. main() function
- 5. cout and
- 6. Built-in types
- 7. Booleans (bool)
- 8. Characters (char)
- 9. Integers (int)
- 10. Floating-point numbers (floatdouble)
- 11. Arithmetic Operators
- 12. Order of Evaluation
- 13. Comparison Operators
- 14. const
- 15. Strings
- 17. Answer and Explanation to Coding Exercise
- 18. Key Takeaways
- 20. Answers and Explanations
3. Input, Conditional Statements & Loops
- 1. Section Introduction
- 2. cin and
- 3. if - else if - else
- 5. Answer and Explanation to Coding Exercise
- 6. switch
- 8. Answer and Explanation to Coding Exercise
- 9. for loops
- 11. Answer and Explanation to Coding Exercise
- 12. while loops
- 14. Answer and Explanation to Coding Exercise
- 15. do-while loops
- 16. goto
- 17. Pointers
- 18. Arrays
- 20. Answer and Explanation to Coding Exercise
- 21. Vectors
- 23. Answer and Explanation to Coding Exercise
- 24. Key Takeaways
- 26. Answers and Explanations
4. User-Defined Types
5. Intermediate C++
- 1. Section Introduction
- 2. Libraries
- 3. The Standard-Library
- 4. File IO
- 5. Date & Time
- 7. Answer and Explanation to Coding Exercise
- 8. Recursion
- 10. Answer and Explanation to Coding Exercise
- 11. Regular Expressions (REGEX)
- 12. Linked Lists
- 14. Answer and Explanation to Coding Exercise
- 15. Trees
- 17. Answer and Explanation to Coding Exercise
- 18. Key Takeaways
- 20. Answers and Explanations
6. Containers
- 1. Section Introduction
- 2. vector
- 4. Answer and Explanation to Coding Exercise
- 5. forward_list
- 7. Answer and Explanation to Coding Exercise
- 8. list
- 10. Answer and Explanation to Coding Exercise
- 11. deque
- 13. Answer and Explanation to Coding Exercise
- 14. set
- 16. Answer and Explanation to Coding Exercise
- 17. map
- 19. Answer and Explanation to Coding Exercise
- 20. stack
- 22. Answer and Explanation to Coding Exercise
- 23. queue
- 25. Answer and Explanation to Coding Exercise
- 26. Key Takeaways
- 28. Answers and Explanations
7. Object-Oriented Programming (OOP)
8. String Algorithms
- 1. Section Introduction
- 2. Roman to Integer
- 4. Answer and Explanation to Coding Exercise
- 5. Palindrome
- 7. Answer and Explanation to Coding Exercise
- 8. Reverse Characters in a Character Array
- 10. Answer and Explanation to Coding Exercise
- 11. Valid Parentheses
- 13. Answer and Explanation to Coding Exercise
- 14. Valid Anagram
- 16. Answer and Explanation to Coding Exercise
- 17. Key Takeaways
9. Array and Vector Algorithms
- 1. Section Introduction
- 2. Remove Element
- 4. Answer and Explanation to Coding Exercise
- 5. Remove Duplicates From Sorted Array
- 7. Answer and Explanation to Coding Exercise
- 8. Pascal's Triangle
- 10. Answer and Explanation to Coding Exercise
- 11. Sort Array By Parity
- 13. Answer and Explanation to Coding Exercise
- 14. Key Takeaways
10. Tree Algorithms
- 1. Section Introduction
- 2. Inorder Traversal
- 4. Preorder Traversal
- 6. Postorder Traversal
- 8. Maximum Depth (Height) of Binary Tree
- 10. Answer and Explanation to Coding Exercise
- 11. Balanced Binary Tree
- 13. Answer and Explanation to Coding Exercise
- 14. Path Sum
- 16. Answer and Explanation to Coding Exercise
- 17. Breadth First Search (BFS)
- 19. Answer and Explanation to Coding Exercise
- 20. Depth First Search (DFS)
- 22. Answer and Explanation to Coding Exercise
- 23. Key Takeaways
11. Linked List Algorithms
12. Course Summary and Wrap-Up
课程内容
12个章节 , 146个讲座
1. Introduction
- 1. Introduction (免费)
- 2. Program Installation & Setup (Mac) (免费)
- 3. Program Installation & Setup (Windows)
- 4. Creating a C++ (.cpp) file
- 5. Hello World in C++
- 6. Overview of the C++ Programming Language
- 8. Answer and Explanation to Coding Exercise
- 9. History of C++
- 10. Running C++ Code on Mac (Terminal)
- 11. Running C++ Code on Windows (Terminal)
- 12. Key Takeaways
2. Basics of C++
- 1. Section Introduction
- 2. How is a C++ program run
- 3. #include
- 4. main() function
- 5. cout and
- 6. Built-in types
- 7. Booleans (bool)
- 8. Characters (char)
- 9. Integers (int)
- 10. Floating-point numbers (floatdouble)
- 11. Arithmetic Operators
- 12. Order of Evaluation
- 13. Comparison Operators
- 14. const
- 15. Strings
- 17. Answer and Explanation to Coding Exercise
- 18. Key Takeaways
- 20. Answers and Explanations
3. Input, Conditional Statements & Loops
- 1. Section Introduction
- 2. cin and
- 3. if - else if - else
- 5. Answer and Explanation to Coding Exercise
- 6. switch
- 8. Answer and Explanation to Coding Exercise
- 9. for loops
- 11. Answer and Explanation to Coding Exercise
- 12. while loops
- 14. Answer and Explanation to Coding Exercise
- 15. do-while loops
- 16. goto
- 17. Pointers
- 18. Arrays
- 20. Answer and Explanation to Coding Exercise
- 21. Vectors
- 23. Answer and Explanation to Coding Exercise
- 24. Key Takeaways
- 26. Answers and Explanations
4. User-Defined Types
5. Intermediate C++
- 1. Section Introduction
- 2. Libraries
- 3. The Standard-Library
- 4. File IO
- 5. Date & Time
- 7. Answer and Explanation to Coding Exercise
- 8. Recursion
- 10. Answer and Explanation to Coding Exercise
- 11. Regular Expressions (REGEX)
- 12. Linked Lists
- 14. Answer and Explanation to Coding Exercise
- 15. Trees
- 17. Answer and Explanation to Coding Exercise
- 18. Key Takeaways
- 20. Answers and Explanations
6. Containers
- 1. Section Introduction
- 2. vector
- 4. Answer and Explanation to Coding Exercise
- 5. forward_list
- 7. Answer and Explanation to Coding Exercise
- 8. list
- 10. Answer and Explanation to Coding Exercise
- 11. deque
- 13. Answer and Explanation to Coding Exercise
- 14. set
- 16. Answer and Explanation to Coding Exercise
- 17. map
- 19. Answer and Explanation to Coding Exercise
- 20. stack
- 22. Answer and Explanation to Coding Exercise
- 23. queue
- 25. Answer and Explanation to Coding Exercise
- 26. Key Takeaways
- 28. Answers and Explanations
7. Object-Oriented Programming (OOP)
8. String Algorithms
- 1. Section Introduction
- 2. Roman to Integer
- 4. Answer and Explanation to Coding Exercise
- 5. Palindrome
- 7. Answer and Explanation to Coding Exercise
- 8. Reverse Characters in a Character Array
- 10. Answer and Explanation to Coding Exercise
- 11. Valid Parentheses
- 13. Answer and Explanation to Coding Exercise
- 14. Valid Anagram
- 16. Answer and Explanation to Coding Exercise
- 17. Key Takeaways
9. Array and Vector Algorithms
- 1. Section Introduction
- 2. Remove Element
- 4. Answer and Explanation to Coding Exercise
- 5. Remove Duplicates From Sorted Array
- 7. Answer and Explanation to Coding Exercise
- 8. Pascal's Triangle
- 10. Answer and Explanation to Coding Exercise
- 11. Sort Array By Parity
- 13. Answer and Explanation to Coding Exercise
- 14. Key Takeaways
10. Tree Algorithms
- 1. Section Introduction
- 2. Inorder Traversal
- 4. Preorder Traversal
- 6. Postorder Traversal
- 8. Maximum Depth (Height) of Binary Tree
- 10. Answer and Explanation to Coding Exercise
- 11. Balanced Binary Tree
- 13. Answer and Explanation to Coding Exercise
- 14. Path Sum
- 16. Answer and Explanation to Coding Exercise
- 17. Breadth First Search (BFS)
- 19. Answer and Explanation to Coding Exercise
- 20. Depth First Search (DFS)
- 22. Answer and Explanation to Coding Exercise
- 23. Key Takeaways