完整的 C 编程课程 - 面向学生的 C 语言
上次更新时间:2024-11-13
课程售价: 2.9 元
联系右侧微信客服充值或购买课程
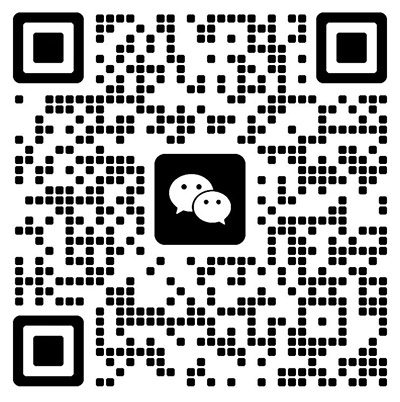
课程内容
1. Welcome to Course!
2. Introduction to General Programming Fundamentals!
3. Environment Setup - Choosing Your IDE
4. Additional IDEs Setup [Optional Section]
- 1. Visual Studio Windows - Download
- 2. Visual Studio Windows - Getting Started
- 3. Visual Studio Code Windows - Download & Install
- 4. Visual Studio Code Windows Official - Getting Started
- 5. Eclipse - Getting Started using C (Windows)
- 6. CodeLite Windows - Download & Install
- 7. CodeLite Windows - Getting Started
5. Welcome First Program [+3 Full Exercises & Video Solutions]
6. Comments & Format Specification
7. ACADEMIC PRACTICE #1 College Exercises & Solutions - Basics C Programming
8. Variables & Data Types - From Zero To Hero!
- 1. Variables Introduction
- 2. Variables - General Concept In Programming
- 3. Variables - Complete Usage in C Language
- 4. Variables Code Example #1
- 5. Challenge #1 - Find your Year of Birth!
- 6. Challenge #1 - Solution
- 7. Challenge #2 - Calculate Rectangle's Area
- 8. Challenge #2 - Solution
- 9. Casting Introduction
- 10. Casting in C Programming Language
- 11. Challenge #3 - Calculating your Average Grade
- 12. Challenge #3 - Solution
- 13. Milestone 2 - Weather Station A
- 14. General SWAP in Programming
- 15. SWAP in C Language
- 16. Variables - Summary! You're doing GREAT!
9. ACADEMIC PRACTICE #2 College Exercises & Solutions - Variables & Data Types
- 1. Arithmetic Sequence - General Introduction and Explanation
- 2. n-th Term of Arithmetic Sequence - Question
- 3. n-th Term of Arithmetic Sequence - Solution
- 4. Sum of Arithmetic Sequence - Question
- 5. Sum of Arithmetic Sequence - Solution
- 6. Employee Salary Calculator - Question
- 7. Employee Salary Calculator - Solution
- 8. Finding a Driving Time - Question
- 9. Finding a Driving Time - Solution
- 10. Convert Seconds into Hours, Minutes, and Seconds - Question
- 11. Convert Seconds into Hours, Minutes, and Seconds - Solution
- 12. Removing Decimal Part from an Integer - Question
- 13. Removing Decimal Part from an Integer - Solution
- 14. Sum of Triple Digits - Question
- 15. Sum of Triple Digits - Solution
- 16. Print Reversed Number - Question
- 17. Print Reversed Number - Solution
- 18. Distance Between 2 Points - Question
- 19. Distance Between 2 Points - Solution
- 20. Efficient Calculation Example - Question
- 21. Efficient Calculation Example - Solution
11. Conditions And Control Flow - Is That TrueFalse
- 1. Control Flow - Introduction
- 2. Congratulations Failed - How should you decide
- 3. Congratulations In C - Complete Usage Of Conditions In C Programming
- 4. Finding Maximum between 2 numbers - General Concept
- 5. Finding Maximum - Implementation in C!
- 6. Control Flow - Relational Operators
- 7. Challenge #1 - Find if number is Odd or Even
- 8. Challenge #1 - Solution
- 9. Challenge #2 - print MIN and MAX
- 10. Challenge #2 - Solution
- 11. Challenge #3 - min & max among 3 numbers
- 12. Challenge #3 - Solution
- 13. Control Flow - Moving Forward!
- 14. Moving Forward - in C Language
- 15. Logical Operators - General Concept
- 16. Logical Operators in C Programming Language
- 17. Switch Case + FULL Example
- 18. Conditions - Summary! Amazing Concept, TrueFalse )
- 19. Milestone - Intermediate Calculator (using Switch Case)
- 20. EXTRA Milestone - Solution
12. ACADEMIC PRACTICE #3 College Exercises & Solutions - Conditions & Control Flow
- 1. Check if Numbers are the same - Question
- 2. Check if Numbers are the same - Solution
- 3. Check if a number is double-digit or triple-digit - Question
- 4. Check if a number is double-digit or triple-digit - Solution
- 5. Print the Absolute Number - Question
- 6. Print the Absolute Number - Solution
- 7. Quadrant of a Point - Question
- 8. Quadrant of a Point - Solution
- 9. Month Number to Month Name - Question
- 10. Month Number to Month Name - Solution
- 11. Unique Clock Representation - Question
- 12. Unique Clock Representation - Solution
- 13. Divisible 3 Numbers Example - Question
- 14. Divisible 3 Numbers Example - Solution
- 15. Is Leap Year - Question
- 16. Is Leap Year - Solution
- 17. Find the next day on Calendar! - Question
- 18. Find the next day on Calendar! - Solution
13. Loops - For, While, And Do-While
- 1. Introduction
- 2. Why using loops
- 3. While Loops - General Structure
- 4. While Loops in C Programming Language!
- 5. Challenge #1 - Printing any number of asterisks
- 6. Challenge #1 - Solution
- 7. Challenge #2 - Adding Pow to Calculator!
- 8. Challenge #2 - Solution
- 9. Milestone #1 - Coolest Average Grade Calculator
- 10. EXTRA Milestone #1 - Solution
- 11. Do-While Loops - C Programming Language
- 12. Challenge #3 - Enter a legal grade!
- 13. Challenge #3 - Solution
- 14. For Loops - General
- 15. For Loops in C Programming Language
- 16. Challenge #4 - Adding POW (using For Loops)
- 17. Challenge #4 - Solution
- 18. Challenge #5! Punishment!
- 19. Challenge #5 - Punishment Solution
- 20. Nested Loops - Print Right Angle Triangle Pattern - part #1 - Question
- 21. Nested Loops - Print Right Angle Triangle Pattern - part #1 - Solution
- 22. Nested Loops - Print Right Angle Triangle Pattern - part #2 - Question
- 23. Nested Loops - Print Right Angle Triangle Pattern - part #2 - Solution
- 24. Milestone #2 - 10 Cents Million Dollars! $$$ (Ka-ching!).
- 25. Milestone #2 - Solution!
14. ACADEMIC PRACTICE #4 College Exercises & Solutions - Loops in C
- 1. Print from 1 to num and vice versa - Question
- 2. Print from 1 to num and vice versa - Solution
- 3. Print the sum of all numbers from 1 to num - Question
- 4. Print the sum of all numbers from 1 to num - Solution
- 5. Print the Multiplication Table of a number - Question
- 6. Print the Multiplication Table of a number - Solution
- 7. Print n even natural numbers - Question
- 8. Print n even natural numbers - Solution
- 9. Print Pyramid Numbers Pattern - Question
- 10. Print Pyramid Numbers Pattern - Solution
- 11. Print Sum of Values Divisible by 3 AND 5 - Trivial & Optimized Solutions - Quest
- 12. Print Sum of Values Divisible by 3 AND 5 - Trivial & Optimized Solutions - Solut
- 13. Print Sum of Values Divisible by 3 OR 5 - Trivial & Optimized Solutions - Quest
- 14. Print Sum of Values Divisible by 3 OR 5 - Trivial & Optimized Solutions - Solut
- 15. Very Ascending or Not - Question
- 16. Very Ascending or Not - Solution
- 17. Subtracting EvenDigitsSum and OddDigitsSum - Solution
- 18. Sum of Odd Numbers Sequence - Solution
15. Functions - Don't Get Scared! It's Kind Of Fun!
- 1. Functions - General Concept
- 2. Functions - Basic Structure
- 3. Functions Usage – in C Programming Language
- 4. Function Example #1 - Print Greetings!
- 5. Function Example #2 - Print Max between 2 Integers
- 6. Function Example #3 - Return Max between 3 Integers
- 7. Function Example #4 - Calc & Return avg between 3 numbers
16. ACADEMIC PRACTICE #5 College Exercises & Solutions - Functions in C
- 1. Challenge #1 - Find Rectangle Area
- 2. Challenge #1 - Solution
- 3. Challenge #2 - Maximum DIGIT in a 2-digits number
- 4. Challenge #2 - Solution
- 5. Challenge #3 - Calculate FACTORIAL!
- 6. Challenge #3 - Solution
- 7. isEven Function C Exercise
- 8. isOdd Function C Exercise
- 9. Sequence of 9s
- 10. Generation of Numbers 123...seq length
- 11. toLower Function in C - Implementation
- 12. toUpper Function in C - Implementation
- 13. Chars To 3 Digits Integer
- 14. Amount And Average Of Digits Less Than Given Digit - Question
- 15. Amount And Average Of Digits Less Than Given Digit - Solution
- 16. Basic Mathematical Question - Derivatives [Optional]
17. Arrays - Concept & 1D Arrays
- 1. Arrays - General
- 2. Arrays – Declaration
- 3. Arrays - Initialization
- 4. Arrays – Element Accessing
- 5. Challenge #1 – Finding Maximum Value in Array
- 6. Challenge #1 - Solution
- 7. Challenge #2 - Finding Maximum Index in Array
- 8. Challenge #2 - Solution
- 9. Using a #define
- 10. Good Multiplication Neighbors
- 11. Challenge #3 Display array values in reverse order
18. Matrix - 2D Arrays
19. Pointers - Wooha!
20. 1D Arrays - Intermediate Level Exercises
- 1. Arrays - Copying an Array - Question
- 2. Arrays - Copying an Array - Solution
- 3. Array - Palindrome Exercise
- 4. Arrays - Largest Neighbors Sum
- 5. A Program to find if an Array is sorted - Question
- 6. A Program to find if an Array is sorted - Solution
- 7. A Program to print and count all unique elements in an array - Question
- 8. A Program to print and count all unique elements in an array - Solution
- 9. A Program to count a total number of “non-unique” values in an array - Question
- 10. A Program to count a total number of “non-unique” values in an array - Solution
- 11. A Program to Rotate Left a given array by 1 positions - Question
- 12. A Program to Rotate Left a given array by 1 positions - Solution
- 13. Continue (Upgrade) Rotate Left a given array by N positions - Question
- 14. Continue (Upgrade) Rotate Left a given array by N positions - Solution
- 15. Continue (Upgrade2) Rotate RIGHT a given array by N positions - Question
- 16. Continue (Upgrade2) Rotate RIGHT a given array by N positions - Solution
- 17. A Program to Find Two elements whose Sum is Closest to Zero - Question
- 18. A Program to Find Two Elements whose Sum is Closest to Zero - Solution
- 19. Calculate Any Array Sum
- 20. Reset All Elements of An Array to 0
- 21. Swap 2 Array Values One-by-One
- 22. SUPER EXERCISE Swap O(1) Complexity!
21. Strings In General And In C Programming
- 1. Strings - General
- 2. Introduction to Strings in C!
- 3. String Initialization
- 4. Challenge #1 - Initialize a String & Print it!
- 5. Challenge #1 - Solution
- 6. Strings - Input & Output
- 7. Challenge #2 - Find Length of String
- 8. Challenge #2 - Solution
- 9. Let's INTRODUCE string.h!
- 10. Challenge #3 - Number of WORDS in a SENTENCE
- 11. Challenge #3 - Solution
- 12. 10. Milestone #1 - PALINDROME MILESTONE!
22. ACADEMIC PRACTICE #7 College Exercises & Solutions - Strings in C
23. Recursions Recursions Recursions!!
- 1. Recursions - General Visualization + Introduction
- 2. Example 1 - Sum of Arithmetical Progression
- 3. Example 1 - Arithmetic Progression - Solution
- 4. Example 2 - Factorial
- 5. Example 2 - Factorial - Solution
- 6. Challenge #1 - Fibonacci
- 7. Challenge #1 - Solution
- 8. Challenge #2 - Sum of Digits in a Number
- 9. Challenge #2 - Solution
- 10. Challenge #3 - Count of Digits
- 11. Challenge #3 - Solution
24. Recursions in C - Academic Practice
- 1. Recursive Function - Total Numbers Less Than Num - Question
- 2. Recursive Function - Total Numbers Less Than Num - Solution
- 3. Total Characters in a Sequence - Question
- 4. Total Characters in a Sequence - Solution
- 5. Total Even Numbers in a Sequence - Question
- 6. Total Even Numbers in a Sequence - Solution
- 7. Sum of All Even Numbers - Question
- 8. Sum of All Even Numbers - Solution
- 9. Printing1ToN and NTo1 - Question
- 10. Printing1ToN and NTo1 - Solution
- 11. Printing NTo1 And Vice Versa (No 1's Duplications)
- 12. Lucas Sequence
- 13. Finding Maximum Value in a Sequence of Elements (Using Recursion)
- 14. Finding Minimum Value in a Sequence of Elements
- 15. Pell Number in a Pell Sequence
- 16. Advanced - Even Digits Sum
- 17. Advanced - Even Digits Sum [OPTIMIZED & MINIMIZED]
- 18. Advanced - Odd Digits Sum
- 19. Advanced - Odd Even Positions and Values Finder - Question
- 20. Advanced - Odd Even Positions and Values Finder - Solution
- 21. Extra Recursion #1 - Print Sequence of num1s and then Sequence of num2s - Quest.
- 22. Extra Recursion #1 - Print Sequence of num1s and then Sequence of num2s - Solut.
- 23. Extra Recursion #2 - Print Sequence of char1s and then Sequencex2 of char2s - Q.
- 24. Extra Recursion #2 - Print Sequence of char1s and then Sequencex2 of char2s - S.
- 25. Extra Recursion #3 - Print Seq. of LowerCase Chars + Seq. of UpperCase Chars - Q
- 26. Extra Recursion #3 - Print Seq. of LowerCase Chars + Seq. of UpperCase Chars - S
- 27. Extra Recursion #4 - Find if Digits are Ascending, Descending, or not - Question
- 28. Extra Recursion #4 - Find if Digits are Ascending, Descending, or not - Solution
- 29. Extra Recursion #5 - Even_Odd Count of Digit Occurrences in num - Question
- 30. Extra Recursion #5 - Even_Odd Count of Digit Occurrences in num - Solution
- 31. Extra Recursion #5 - Minimized and Optimized Solution
25. Structs - Additional Content in C Programming
- 1. Structures - Intro
- 2. Creating a Structure Template
- 3. Structure Variables
- 4. Challenge #1 - Initialize & Print Date Variable
- 5. Typedef & Structures
- 6. Challenge #4 - General Function to use Input & Print of a struct
- 7. Challenge #5 - Find the Next Date
- 8. Initializing a Struct in C
- 9. Exercise - Input Point & Print Point - Question
- 10. Exercise - Input Point & Print Point - Solution
- 11. Static Array of Structs
- 12. Structs having arrays as data members (and copying struct variables)
- 13. Logical Operators #1 - Can we use Relational Operators on Structs by default
- 14. Logical Operators #2 - Writing Associate Logical Functions
- 15. Mathematical Operators - Writing Associate Functions
- 16. Comprehensive Exercise - Rational Numbers Struct - Question
- 17. Comprehensive Exercise - Rational Numbers Struct - Solution
- 18. Composing struct inside another struct
- 19. Structs as the BASIS for more Advanced Topics
26. Unions - Additional Content C Programming Language
27. Constants
28. Extra [Optional] - Working with FILES!
- 1. General Introduction to Files
- 2. What is a file [as a stream of bytes]
- 3. Examples of Stream already used (InputOutputError)
- 4. Start with Files Steps and Syntax in C
- 5. Creating a File and Reading from a File
- 6. Intro to 6 basic functions for working with textual files
- 7. fgetc - function
- 8. fputc - function
- 9. fprintf & fscanf - functions
- 10. fputs - function
- 11. fgets - function
- 12. Introducing EOF (End of File)
- 13. Exercise #1 - Number of characters in a file [Question]
- 14. Exercise #1 - Number of characters in a file [Solution]
- 15. Exercise #2 - Number of Lines in a file [Question]
- 16. Exercise #2 - Number of Lines in a file [Solution]
- 17. Exercise #3 - Writing numbers and their powers to a file[Question]
- 18. Exercise #3 - Writing numbers and their powers to a file[Solution]
- 19. Exercise #4 - Reading numbers from a file [Question]
- 20. Exercise #4 - Reading numbers from a file [Solution]
- 21. Exercise #5 - A program to calculate character appearances in a file - Question
- 22. Exercise #5 - A program to calculate character appearances in a file - Solution
- 23. Exercise #6 - lowerFrequencyAppearances Program for Lowercase Letters - Question
- 24. Exercise #6 - lowerFrequencyAppearances Program for Lowercase Letters - Solution
- 25. Exercise #7 - Print Max Appearances LowerCase Letter in File - Question
- 26. Exercise #7 - Print Max Appearances LowerCase Letter in File - Solution
29. Extra [Optional] - Linked Lists Practice!
课程内容
29个章节 , 340个讲座
1. Welcome to Course!
2. Introduction to General Programming Fundamentals!
3. Environment Setup - Choosing Your IDE
4. Additional IDEs Setup [Optional Section]
- 1. Visual Studio Windows - Download
- 2. Visual Studio Windows - Getting Started
- 3. Visual Studio Code Windows - Download & Install
- 4. Visual Studio Code Windows Official - Getting Started
- 5. Eclipse - Getting Started using C (Windows)
- 6. CodeLite Windows - Download & Install
- 7. CodeLite Windows - Getting Started
5. Welcome First Program [+3 Full Exercises & Video Solutions]
6. Comments & Format Specification
7. ACADEMIC PRACTICE #1 College Exercises & Solutions - Basics C Programming
8. Variables & Data Types - From Zero To Hero!
- 1. Variables Introduction
- 2. Variables - General Concept In Programming
- 3. Variables - Complete Usage in C Language
- 4. Variables Code Example #1
- 5. Challenge #1 - Find your Year of Birth!
- 6. Challenge #1 - Solution
- 7. Challenge #2 - Calculate Rectangle's Area
- 8. Challenge #2 - Solution
- 9. Casting Introduction
- 10. Casting in C Programming Language
- 11. Challenge #3 - Calculating your Average Grade
- 12. Challenge #3 - Solution
- 13. Milestone 2 - Weather Station A
- 14. General SWAP in Programming
- 15. SWAP in C Language
- 16. Variables - Summary! You're doing GREAT!
9. ACADEMIC PRACTICE #2 College Exercises & Solutions - Variables & Data Types
- 1. Arithmetic Sequence - General Introduction and Explanation
- 2. n-th Term of Arithmetic Sequence - Question
- 3. n-th Term of Arithmetic Sequence - Solution
- 4. Sum of Arithmetic Sequence - Question
- 5. Sum of Arithmetic Sequence - Solution
- 6. Employee Salary Calculator - Question
- 7. Employee Salary Calculator - Solution
- 8. Finding a Driving Time - Question
- 9. Finding a Driving Time - Solution
- 10. Convert Seconds into Hours, Minutes, and Seconds - Question
- 11. Convert Seconds into Hours, Minutes, and Seconds - Solution
- 12. Removing Decimal Part from an Integer - Question
- 13. Removing Decimal Part from an Integer - Solution
- 14. Sum of Triple Digits - Question
- 15. Sum of Triple Digits - Solution
- 16. Print Reversed Number - Question
- 17. Print Reversed Number - Solution
- 18. Distance Between 2 Points - Question
- 19. Distance Between 2 Points - Solution
- 20. Efficient Calculation Example - Question
- 21. Efficient Calculation Example - Solution
11. Conditions And Control Flow - Is That TrueFalse
- 1. Control Flow - Introduction
- 2. Congratulations Failed - How should you decide
- 3. Congratulations In C - Complete Usage Of Conditions In C Programming
- 4. Finding Maximum between 2 numbers - General Concept
- 5. Finding Maximum - Implementation in C!
- 6. Control Flow - Relational Operators
- 7. Challenge #1 - Find if number is Odd or Even
- 8. Challenge #1 - Solution
- 9. Challenge #2 - print MIN and MAX
- 10. Challenge #2 - Solution
- 11. Challenge #3 - min & max among 3 numbers
- 12. Challenge #3 - Solution
- 13. Control Flow - Moving Forward!
- 14. Moving Forward - in C Language
- 15. Logical Operators - General Concept
- 16. Logical Operators in C Programming Language
- 17. Switch Case + FULL Example
- 18. Conditions - Summary! Amazing Concept, TrueFalse )
- 19. Milestone - Intermediate Calculator (using Switch Case)
- 20. EXTRA Milestone - Solution
12. ACADEMIC PRACTICE #3 College Exercises & Solutions - Conditions & Control Flow
- 1. Check if Numbers are the same - Question
- 2. Check if Numbers are the same - Solution
- 3. Check if a number is double-digit or triple-digit - Question
- 4. Check if a number is double-digit or triple-digit - Solution
- 5. Print the Absolute Number - Question
- 6. Print the Absolute Number - Solution
- 7. Quadrant of a Point - Question
- 8. Quadrant of a Point - Solution
- 9. Month Number to Month Name - Question
- 10. Month Number to Month Name - Solution
- 11. Unique Clock Representation - Question
- 12. Unique Clock Representation - Solution
- 13. Divisible 3 Numbers Example - Question
- 14. Divisible 3 Numbers Example - Solution
- 15. Is Leap Year - Question
- 16. Is Leap Year - Solution
- 17. Find the next day on Calendar! - Question
- 18. Find the next day on Calendar! - Solution
13. Loops - For, While, And Do-While
- 1. Introduction
- 2. Why using loops
- 3. While Loops - General Structure
- 4. While Loops in C Programming Language!
- 5. Challenge #1 - Printing any number of asterisks
- 6. Challenge #1 - Solution
- 7. Challenge #2 - Adding Pow to Calculator!
- 8. Challenge #2 - Solution
- 9. Milestone #1 - Coolest Average Grade Calculator
- 10. EXTRA Milestone #1 - Solution
- 11. Do-While Loops - C Programming Language
- 12. Challenge #3 - Enter a legal grade!
- 13. Challenge #3 - Solution
- 14. For Loops - General
- 15. For Loops in C Programming Language
- 16. Challenge #4 - Adding POW (using For Loops)
- 17. Challenge #4 - Solution
- 18. Challenge #5! Punishment!
- 19. Challenge #5 - Punishment Solution
- 20. Nested Loops - Print Right Angle Triangle Pattern - part #1 - Question
- 21. Nested Loops - Print Right Angle Triangle Pattern - part #1 - Solution
- 22. Nested Loops - Print Right Angle Triangle Pattern - part #2 - Question
- 23. Nested Loops - Print Right Angle Triangle Pattern - part #2 - Solution
- 24. Milestone #2 - 10 Cents Million Dollars! $$$ (Ka-ching!).
- 25. Milestone #2 - Solution!
14. ACADEMIC PRACTICE #4 College Exercises & Solutions - Loops in C
- 1. Print from 1 to num and vice versa - Question
- 2. Print from 1 to num and vice versa - Solution
- 3. Print the sum of all numbers from 1 to num - Question
- 4. Print the sum of all numbers from 1 to num - Solution
- 5. Print the Multiplication Table of a number - Question
- 6. Print the Multiplication Table of a number - Solution
- 7. Print n even natural numbers - Question
- 8. Print n even natural numbers - Solution
- 9. Print Pyramid Numbers Pattern - Question
- 10. Print Pyramid Numbers Pattern - Solution
- 11. Print Sum of Values Divisible by 3 AND 5 - Trivial & Optimized Solutions - Quest
- 12. Print Sum of Values Divisible by 3 AND 5 - Trivial & Optimized Solutions - Solut
- 13. Print Sum of Values Divisible by 3 OR 5 - Trivial & Optimized Solutions - Quest
- 14. Print Sum of Values Divisible by 3 OR 5 - Trivial & Optimized Solutions - Solut
- 15. Very Ascending or Not - Question
- 16. Very Ascending or Not - Solution
- 17. Subtracting EvenDigitsSum and OddDigitsSum - Solution
- 18. Sum of Odd Numbers Sequence - Solution
15. Functions - Don't Get Scared! It's Kind Of Fun!
- 1. Functions - General Concept
- 2. Functions - Basic Structure
- 3. Functions Usage – in C Programming Language
- 4. Function Example #1 - Print Greetings!
- 5. Function Example #2 - Print Max between 2 Integers
- 6. Function Example #3 - Return Max between 3 Integers
- 7. Function Example #4 - Calc & Return avg between 3 numbers
16. ACADEMIC PRACTICE #5 College Exercises & Solutions - Functions in C
- 1. Challenge #1 - Find Rectangle Area
- 2. Challenge #1 - Solution
- 3. Challenge #2 - Maximum DIGIT in a 2-digits number
- 4. Challenge #2 - Solution
- 5. Challenge #3 - Calculate FACTORIAL!
- 6. Challenge #3 - Solution
- 7. isEven Function C Exercise
- 8. isOdd Function C Exercise
- 9. Sequence of 9s
- 10. Generation of Numbers 123...seq length
- 11. toLower Function in C - Implementation
- 12. toUpper Function in C - Implementation
- 13. Chars To 3 Digits Integer
- 14. Amount And Average Of Digits Less Than Given Digit - Question
- 15. Amount And Average Of Digits Less Than Given Digit - Solution
- 16. Basic Mathematical Question - Derivatives [Optional]
17. Arrays - Concept & 1D Arrays
- 1. Arrays - General
- 2. Arrays – Declaration
- 3. Arrays - Initialization
- 4. Arrays – Element Accessing
- 5. Challenge #1 – Finding Maximum Value in Array
- 6. Challenge #1 - Solution
- 7. Challenge #2 - Finding Maximum Index in Array
- 8. Challenge #2 - Solution
- 9. Using a #define
- 10. Good Multiplication Neighbors
- 11. Challenge #3 Display array values in reverse order
18. Matrix - 2D Arrays
19. Pointers - Wooha!
20. 1D Arrays - Intermediate Level Exercises
- 1. Arrays - Copying an Array - Question
- 2. Arrays - Copying an Array - Solution
- 3. Array - Palindrome Exercise
- 4. Arrays - Largest Neighbors Sum
- 5. A Program to find if an Array is sorted - Question
- 6. A Program to find if an Array is sorted - Solution
- 7. A Program to print and count all unique elements in an array - Question
- 8. A Program to print and count all unique elements in an array - Solution
- 9. A Program to count a total number of “non-unique” values in an array - Question
- 10. A Program to count a total number of “non-unique” values in an array - Solution
- 11. A Program to Rotate Left a given array by 1 positions - Question
- 12. A Program to Rotate Left a given array by 1 positions - Solution
- 13. Continue (Upgrade) Rotate Left a given array by N positions - Question
- 14. Continue (Upgrade) Rotate Left a given array by N positions - Solution
- 15. Continue (Upgrade2) Rotate RIGHT a given array by N positions - Question
- 16. Continue (Upgrade2) Rotate RIGHT a given array by N positions - Solution
- 17. A Program to Find Two elements whose Sum is Closest to Zero - Question
- 18. A Program to Find Two Elements whose Sum is Closest to Zero - Solution
- 19. Calculate Any Array Sum
- 20. Reset All Elements of An Array to 0
- 21. Swap 2 Array Values One-by-One
- 22. SUPER EXERCISE Swap O(1) Complexity!
21. Strings In General And In C Programming
- 1. Strings - General
- 2. Introduction to Strings in C!
- 3. String Initialization
- 4. Challenge #1 - Initialize a String & Print it!
- 5. Challenge #1 - Solution
- 6. Strings - Input & Output
- 7. Challenge #2 - Find Length of String
- 8. Challenge #2 - Solution
- 9. Let's INTRODUCE string.h!
- 10. Challenge #3 - Number of WORDS in a SENTENCE
- 11. Challenge #3 - Solution
- 12. 10. Milestone #1 - PALINDROME MILESTONE!
22. ACADEMIC PRACTICE #7 College Exercises & Solutions - Strings in C
23. Recursions Recursions Recursions!!
- 1. Recursions - General Visualization + Introduction
- 2. Example 1 - Sum of Arithmetical Progression
- 3. Example 1 - Arithmetic Progression - Solution
- 4. Example 2 - Factorial
- 5. Example 2 - Factorial - Solution
- 6. Challenge #1 - Fibonacci
- 7. Challenge #1 - Solution
- 8. Challenge #2 - Sum of Digits in a Number
- 9. Challenge #2 - Solution
- 10. Challenge #3 - Count of Digits
- 11. Challenge #3 - Solution
24. Recursions in C - Academic Practice
- 1. Recursive Function - Total Numbers Less Than Num - Question
- 2. Recursive Function - Total Numbers Less Than Num - Solution
- 3. Total Characters in a Sequence - Question
- 4. Total Characters in a Sequence - Solution
- 5. Total Even Numbers in a Sequence - Question
- 6. Total Even Numbers in a Sequence - Solution
- 7. Sum of All Even Numbers - Question
- 8. Sum of All Even Numbers - Solution
- 9. Printing1ToN and NTo1 - Question
- 10. Printing1ToN and NTo1 - Solution
- 11. Printing NTo1 And Vice Versa (No 1's Duplications)
- 12. Lucas Sequence
- 13. Finding Maximum Value in a Sequence of Elements (Using Recursion)
- 14. Finding Minimum Value in a Sequence of Elements
- 15. Pell Number in a Pell Sequence
- 16. Advanced - Even Digits Sum
- 17. Advanced - Even Digits Sum [OPTIMIZED & MINIMIZED]
- 18. Advanced - Odd Digits Sum
- 19. Advanced - Odd Even Positions and Values Finder - Question
- 20. Advanced - Odd Even Positions and Values Finder - Solution
- 21. Extra Recursion #1 - Print Sequence of num1s and then Sequence of num2s - Quest.
- 22. Extra Recursion #1 - Print Sequence of num1s and then Sequence of num2s - Solut.
- 23. Extra Recursion #2 - Print Sequence of char1s and then Sequencex2 of char2s - Q.
- 24. Extra Recursion #2 - Print Sequence of char1s and then Sequencex2 of char2s - S.
- 25. Extra Recursion #3 - Print Seq. of LowerCase Chars + Seq. of UpperCase Chars - Q
- 26. Extra Recursion #3 - Print Seq. of LowerCase Chars + Seq. of UpperCase Chars - S
- 27. Extra Recursion #4 - Find if Digits are Ascending, Descending, or not - Question
- 28. Extra Recursion #4 - Find if Digits are Ascending, Descending, or not - Solution
- 29. Extra Recursion #5 - Even_Odd Count of Digit Occurrences in num - Question
- 30. Extra Recursion #5 - Even_Odd Count of Digit Occurrences in num - Solution
- 31. Extra Recursion #5 - Minimized and Optimized Solution
25. Structs - Additional Content in C Programming
- 1. Structures - Intro
- 2. Creating a Structure Template
- 3. Structure Variables
- 4. Challenge #1 - Initialize & Print Date Variable
- 5. Typedef & Structures
- 6. Challenge #4 - General Function to use Input & Print of a struct
- 7. Challenge #5 - Find the Next Date
- 8. Initializing a Struct in C
- 9. Exercise - Input Point & Print Point - Question
- 10. Exercise - Input Point & Print Point - Solution
- 11. Static Array of Structs
- 12. Structs having arrays as data members (and copying struct variables)
- 13. Logical Operators #1 - Can we use Relational Operators on Structs by default
- 14. Logical Operators #2 - Writing Associate Logical Functions
- 15. Mathematical Operators - Writing Associate Functions
- 16. Comprehensive Exercise - Rational Numbers Struct - Question
- 17. Comprehensive Exercise - Rational Numbers Struct - Solution
- 18. Composing struct inside another struct
- 19. Structs as the BASIS for more Advanced Topics
26. Unions - Additional Content C Programming Language
27. Constants
28. Extra [Optional] - Working with FILES!
- 1. General Introduction to Files
- 2. What is a file [as a stream of bytes]
- 3. Examples of Stream already used (InputOutputError)
- 4. Start with Files Steps and Syntax in C
- 5. Creating a File and Reading from a File
- 6. Intro to 6 basic functions for working with textual files
- 7. fgetc - function
- 8. fputc - function
- 9. fprintf & fscanf - functions
- 10. fputs - function
- 11. fgets - function
- 12. Introducing EOF (End of File)
- 13. Exercise #1 - Number of characters in a file [Question]
- 14. Exercise #1 - Number of characters in a file [Solution]
- 15. Exercise #2 - Number of Lines in a file [Question]
- 16. Exercise #2 - Number of Lines in a file [Solution]
- 17. Exercise #3 - Writing numbers and their powers to a file[Question]
- 18. Exercise #3 - Writing numbers and their powers to a file[Solution]
- 19. Exercise #4 - Reading numbers from a file [Question]
- 20. Exercise #4 - Reading numbers from a file [Solution]
- 21. Exercise #5 - A program to calculate character appearances in a file - Question
- 22. Exercise #5 - A program to calculate character appearances in a file - Solution
- 23. Exercise #6 - lowerFrequencyAppearances Program for Lowercase Letters - Question
- 24. Exercise #6 - lowerFrequencyAppearances Program for Lowercase Letters - Solution
- 25. Exercise #7 - Print Max Appearances LowerCase Letter in File - Question
- 26. Exercise #7 - Print Max Appearances LowerCase Letter in File - Solution