计算机编程简介 - 使用 JAVA
上次更新时间:2024-11-01
课程售价: 2.9 元
联系右侧微信客服充值或购买课程
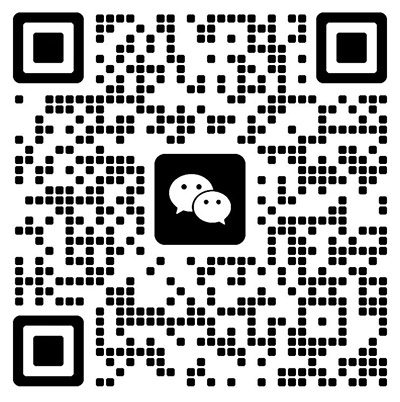
课程内容
- 1. First Java Programming in Eclipse IDE. (免费)
- 2. Keywords, Identifier and Literals. (免费)
- 3. Data Types.
- 4. Type Casting and Auto promotion.
- 5. ASCII, UNICODE and Escape Sequence.
- 6. Strings
- 7. Input through Scanner Class.
- 8. A Program to Compute the area of a Circle
- 9. Celsius to Fahrenheit Conversion
- 10. Pass to Command Line arguments and display a Random between them
- 11. Generate 5 Random Numbers
- 12. Generate 3 Random Characters
- 13. if-else Statements
- 14. Input a number and check whether that is positive, negative or zero
- 15. Input a year and check whether a year is leap or not
- 16. switch-case statements
- 17. check whether a character is uppercase, lowercase, digit or special symbols
- 18. input a character and check whether that is vowel or not
- 19. Generate a Random month between January to December
- 20. while loop and do while loop
- 21. Count the number of digits present in a number
- 22. Find the sum of all the digits of a number
- 23. Print the reverse of a number
- 24. Check whether a number is Armstrong or not
- 25. Check whether a number is Perfect number or not
- 26. Print n number Fibonacci number
- 27. Convert Decimal to Binary
- 28. Print the lcm of 2 numbers
- 29. Add n numbers as per user enter the choice to input
- 30. for Loops
- 31. Generate a Series based on input n1, n2 and n3
- 32. Find the factorial of a number
- 33. Print the multiplication table of a number
- 34. Display n random number and find the average based on the command line arguments
- 35. Check whether a number is Prime or not
- 36. Print the reverse of a String
- 37. Count the number of Vowels present in a String
- 38. Print a list of patterns by simple modification of the code
- 39. Arrays 1D
- 40. Arrays 2D
- 41. Generate 10 Random numbers in an Array
- 42. Count the number of even number present in an array
- 43. Print all the prime number present in an integer array
- 44. Searching an element in an Array
- 45. Inserting an element in an Array
- 46. Deletion of an element in an Array
- 47. Find the smallest element in an Array
- 48. Find the second largest element in any Array
- 49. Transpose a matrix
- 50. Add the elements of columns of a matrix and store in the last row
- 51. Method Introduction, return type, arguments etc.
- 52. Overloading, Recursion and other
- 53. write a method max to find the greater between 2 numbers
- 54. Create a user defined method power to compute X raised to the power n
- 55. A method to count the number of occurrence of a character in a s String
- 56. Calculate the area of a square, circle and rectangle using Method Overloading
- 57. A recursive method to return a factorial of a number
- 58. A recursive method to calculate the sum of the array elements
课程内容
58个讲座
- 1. First Java Programming in Eclipse IDE. (免费)
- 2. Keywords, Identifier and Literals. (免费)
- 3. Data Types.
- 4. Type Casting and Auto promotion.
- 5. ASCII, UNICODE and Escape Sequence.
- 6. Strings
- 7. Input through Scanner Class.
- 8. A Program to Compute the area of a Circle
- 9. Celsius to Fahrenheit Conversion
- 10. Pass to Command Line arguments and display a Random between them
- 11. Generate 5 Random Numbers
- 12. Generate 3 Random Characters
- 13. if-else Statements
- 14. Input a number and check whether that is positive, negative or zero
- 15. Input a year and check whether a year is leap or not
- 16. switch-case statements
- 17. check whether a character is uppercase, lowercase, digit or special symbols
- 18. input a character and check whether that is vowel or not
- 19. Generate a Random month between January to December
- 20. while loop and do while loop
- 21. Count the number of digits present in a number
- 22. Find the sum of all the digits of a number
- 23. Print the reverse of a number
- 24. Check whether a number is Armstrong or not
- 25. Check whether a number is Perfect number or not
- 26. Print n number Fibonacci number
- 27. Convert Decimal to Binary
- 28. Print the lcm of 2 numbers
- 29. Add n numbers as per user enter the choice to input
- 30. for Loops
- 31. Generate a Series based on input n1, n2 and n3
- 32. Find the factorial of a number
- 33. Print the multiplication table of a number
- 34. Display n random number and find the average based on the command line arguments
- 35. Check whether a number is Prime or not
- 36. Print the reverse of a String
- 37. Count the number of Vowels present in a String
- 38. Print a list of patterns by simple modification of the code
- 39. Arrays 1D
- 40. Arrays 2D
- 41. Generate 10 Random numbers in an Array
- 42. Count the number of even number present in an array
- 43. Print all the prime number present in an integer array
- 44. Searching an element in an Array
- 45. Inserting an element in an Array
- 46. Deletion of an element in an Array
- 47. Find the smallest element in an Array
- 48. Find the second largest element in any Array
- 49. Transpose a matrix
- 50. Add the elements of columns of a matrix and store in the last row
- 51. Method Introduction, return type, arguments etc.
- 52. Overloading, Recursion and other
- 53. write a method max to find the greater between 2 numbers
- 54. Create a user defined method power to compute X raised to the power n
- 55. A method to count the number of occurrence of a character in a s String
- 56. Calculate the area of a square, circle and rectangle using Method Overloading
- 57. A recursive method to return a factorial of a number
- 58. A recursive method to calculate the sum of the array elements